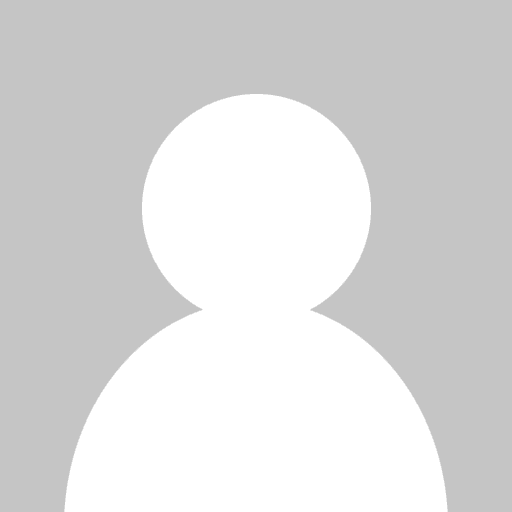
Using Tick History REST API in R language - Part 1
Last Update: June 2021
This is the first article of the educational series that shows you how to use the httr package to retrieve data from the LSEG Tick History REST APIs in R. For this article, we are going to look at the user authentication and get user information. The functions that we are going to use are GET and POST.
Before we start, you are encouraged to visit the REST API Reference tree at the REST API Help Home Page as a primary source of reference for all API functions and supported conditions. You can also view the full list of functions of the httr package here.
The R script for this article is available on GitHub.
User Authentication
Calling the LSEG Tick History REST API to obtain user authentication involves making an HTTP POST request to the LSEG Tick History server. If the call succeeds (i.e. you supply the correct username and password), you will receive an authentication token that must be used for further requests.
The httr package is a wrapper for curl that provides functions for most important http verbs. And since the LSEG Tick History REST API uses JSON as content-type, we will need the jsonlite package as well. Once you’ve installed both packages into your R environment, getting data from the LSEG Tick History REST API is straightforward.
To install the packages use the following command in R:
install.packages('httr','jsonlite')
To load the packages, use:
library(httr)
library(jsonlite)
Next, we need to know the request format. You can check the REST API Reference Tree on API Help Page for an example. Here is the request example in HTTP request form.
POST https://selectapi.datascope.refinitiv.com/RestApi/v1/Authentication/RequestToken
Prefer: respond-async
Content-Type: application/json; odata=minimalmetadata
{
"Credentials": {
"Username": "<Your Username>",
"Password": "<Your Password>"
}
}
Which can translate to httr as follows.
url <- "https://selectapi.datascope.refinitiv.com/RestApi/v1/Authentication/RequestToken"
b <- '{"Credentials":{"Username":"<Your Username>","Password":"<Your Password>"}}'
r <- httr::POST(url,add_headers(prefer = "respond-async"),content_type_json(),body = b)
The above example uses a JSON string as request body, which is easy but not flexible. If you want to change the username and password easily, you may want to use variables instead.
url <- "https://selectapi.datascope.refinitiv.com/RestApi/v1/Authentication/RequestToken"
uname <- '<Your Username>'
pword <- '<Your Password>'
b <- list(Credentials=list(Username=jsonlite::unbox(uname),Password=jsonlite::unbox(pword)))
r <- httr::POST(url,add_headers(prefer = "respond-async"),content_type_json(),body = b,encode = "json")
A successful request will result in a 200 (OK) status. By default, httr::POST will not throw a warning or raise an error if a request did not succeed. You have to use one of the following functions to make sure you find out about HTTP errors.
warn_for_status(r)
stop_for_status(r)
For this article, we will use stop_for_status so that the function stops when there is an error.
Now that we have a response object from a request, we want to extract only the authentication token from the response. We use httr::content to extract content from the response and then use paste to create an authentication string.
a <- httr::content(r, "parsed", "application/json", encoding="UTF-8")
auth <- paste('Token',a[[2]],sep=" ")
The final code should look like this.
library(jsonlite)
library(httr)
url <- "https://selectapi.datascope.refinitiv.com/RestApi/v1/Authentication/RequestToken"
uname <- '<Your Username>'
pword <- '<Your Password>'
b <- list(Credentials=list(Username=jsonlite::unbox(uname),Password=jsonlite::unbox(pword)))
r <- httr::POST(url,add_headers(prefer = "respond-async"),content_type_json(),body = b,encode = "json")
stop_for_status(r)
a <- httr::content(r, "parsed", "application/json", encoding="UTF-8")
auth <- paste('Token',a[[2]],sep=" ")
The authentication token should look like this.
[1] "Token _cQoPNNhx2KvmTKkoW9o7pI7zW9tPotLRFoN_yapOZMKZmc57RPVMCFAKETOKENVSYpGtH9Hycz35nxlfp2GNiCaJzTL6bhoyK_9kU6kN83SuR0LOKPLEASEIGNOREk0T8XoYg5VYfH-iY9H3klCvRr4fylRo2kwW0vP1uUD-eHcT1LFR1eyDxwADgPSRlbUkHNkMk7wXGutxDONOTUSEL9RSHcm14wvPTxhCZndK-HXe_XHeLkGWUUFkrpdDwPkmlDwt7KbL74hvpiuebK4-64EcrlWclCwfXudSSdPs1ns"
Request User Information
Now that we have the authentication token, we shall try requesting your user information. Each HTTP request after the first user authentication must include the authentication token in the header.
Header name='Authorization'
Header value='Token {insert returned token here}'
Authentication tokens expire after 24 hours. Using an expired token will result in a 401 status and require re-authentication.
First, lets us check the example HTTP request form
GET https://selectapi.datascope.refinitiv.com/RestApi/v1/Users/Users
Authorization: Token <your_auth_token_goes_here>
Prefer: respond-async
We created the authentication string in the previous step. To request user information we just use GET
url <- "https://selectapi.datascope.refinitiv.com/RestApi/v1/Users/Users(<Your Username>)"
r <- GET(url,add_headers(prefer = "respond-async",Authorization = auth))
stop_for_status(r)
a <- content(r, "parsed", "application/json", encoding="UTF-8")
Finally, you get a list object containing the UserId, UserName, Email, and Phone.
$`@odata.context`
[1] "https://selectapi.datascope.refinitiv.com/RestApi/v1/$metadata#Users/$entity"
$UserId [1]
9000000
$UserName
[1] "John Doe"
$Email
[1] "john.doe@refinitiv.com"
$Phone
[1] ""
R Script
To make things simpler, we can turn the above code into functions.
library(jsonlite)
library(httr)
RTHLogin <- function(uname,pword) {
url <- "https://selectapi.datascope.refinitiv.com/RestApi/v1/Authentication/RequestToken"
b <- list(Credentials=list(Username=jsonlite::unbox(uname),Password=jsonlite::unbox(pword)))
r <- httr::POST(url,add_headers(prefer = "respond-async"),content_type_json(),body = b,encode = "json")
stop_for_status(r)
a <- httr::content(r, "parsed", "application/json", encoding="UTF-8")
token <- paste('Token',a[[2]],sep=" ")
return(token)
}
RTHUserInfo <- function(token,uname) {
url <- paste0("https://selectapi.datascope.refinitiv.com/RestApi/v1/Users/Users(",uname,")")
r <- GET(url,add_headers(prefer = "respond-async",Authorization = token))
stop_for_status(r)
a<-content(r, "parsed", "application/json", encoding="UTF-8")
return(a)
}
Save the above code as an R script and now we can login to LSEG Tick History by simply calling source("RTH.R") then RTHLogin('Your Username','Your Password')
This concludes the first article. In the next article, we shall look at how to retrieve a user package delivery.