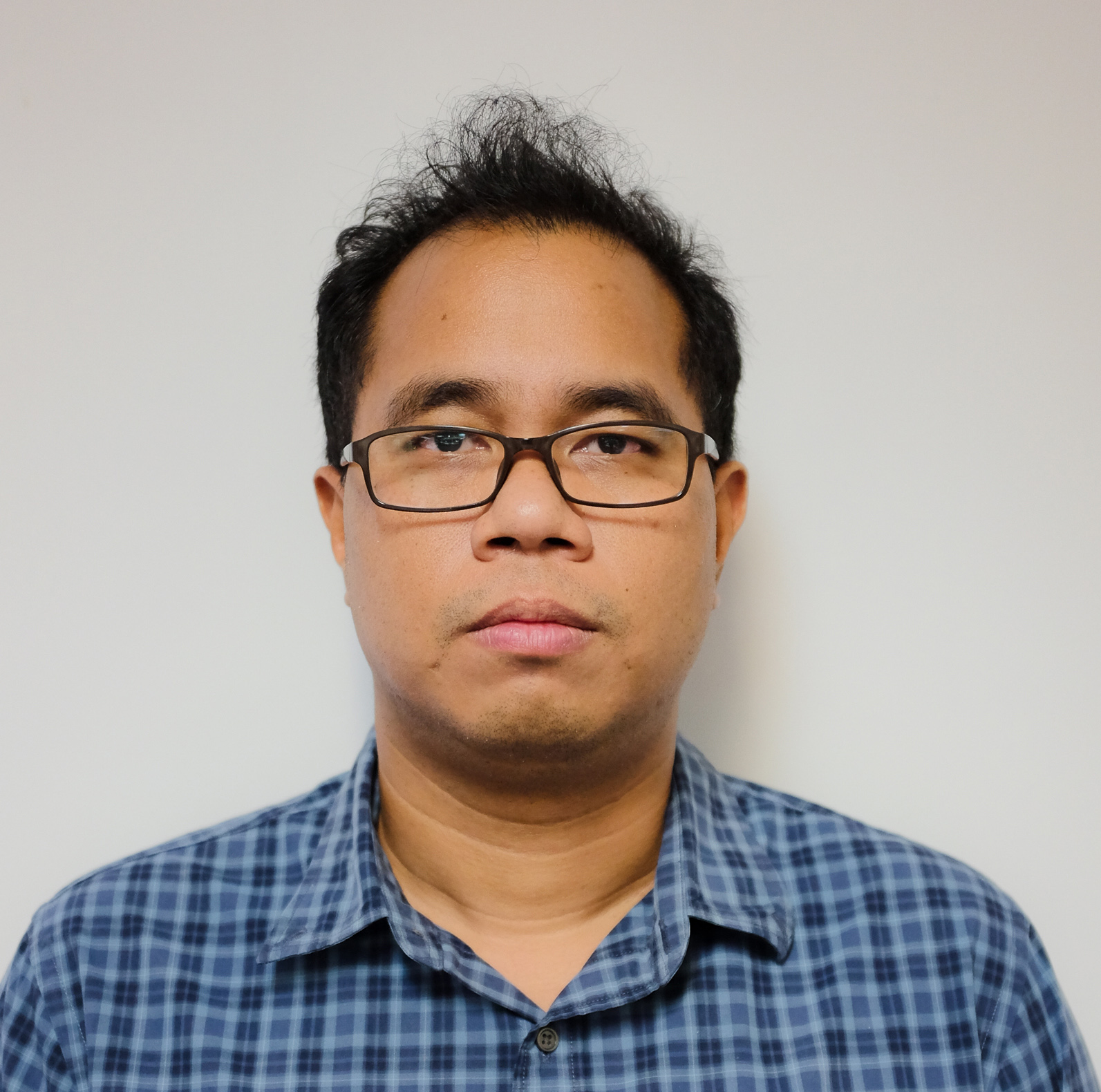
How to implement RKD JSON application with Python chapter 1: the basic
Overview
The Refinitiv Knowledge Direct (RKD) API (formerly known as TRKD API) integrates into your website, trading platform, company intranet/extranet, advisory portal and mobile applications to provide up-to-date financial market data, news and analytics and powerful investment tools.
RKD offers a wide range of Refinitiv' information and services delivered in a request-response scenario via web services using today's industry standard protocols (SOAP/XML and REST/JSON). Connectivity can be via HTTP and HTTPS, over the Internet or Delivery Direct. All data are snapshot (non-streaming) data.
With new HTTP JSON services, developers can integrate RKD information to their application easier then before. This article provides an information regarding the basic knowledge of how to implement RKD JSON application with Python language.
RKD JSON application implementation process
The JSON application requires the following steps to consume data from RKD API services
- Authentication with RKD Authentication service to get an authen token
- Send a request message with the required input information and authen token to the interested RKD service
Authentication Process
The consumer application needs to authenticate with RKD Service Token with the following information
- Username
- Application ID (AppID)
- Password
Once the application is permissioned success, the application gets the following information from RKD
- Token: an encrypted, expiring string that securely identifies the service user (aka service Token)
- Expiration: Token expires after a configurable time period. The default expiration is 90 minutes.
Subscribe Data Process
After the application get the Service Token, the application can subscribes data to the RKD service. The application needs to set the Application ID and service Token with the request message HTTP header to identify the permissioned.
- X-Trkd-Auth-ApplicationID: Application ID
- X-Trkd-Auth-Token: service Token
Data Format
All request and response messages of the RKD REST Services are in the JSON format. We will use the Python json library to encode the request message via the HTTP Post request. For the response message, we will use the JSON builtin encoder of the requests library to decode incoming JSON data.
{
"CreateServiceToken_Response_1": {
"Expiration": "2017-05-09T08:55:52.6017Z",
"Token": "AAA"
}
}
example 1: Example JSON message response for RKD Authenticacion CreateServiceToken_1 service
"trkd_authen.py" Example Application
RKD API uses an encrypted, expiring token to authenticate each request. To create this token, your application submits credentials to the Token Management service. The Token Management service authenticates the credentials and returns the token.
This section describes how to implement the trkd_authen.py script that performs authentication with the TRDK API with the inputted username, password and appid via the command line.
RKD Sservice Token Detail
RKD Service Token URL and Header
The URL enponint for the RKD Service Token is following: https://api.rkd.refinitiv.com/api/TokenManagement/TokenManagement.svc/REST/Anonymous/TokenManagement_1/CreateServiceToken_1
Header:
- Content-type = application/json;charset=utf-8
Method:
- Post
RKD Service TOKEN Request Message
The CreateServiceToken_1 operation requires the following information to perform authentication
- ApplicationID
- Username
- Password
The request message structure is following
{
“CreateServiceToken_Request_1”: {
“ApplicationID”: <application id>,
“Username”: <username>,
“Password”: <password>
}
}
The example of the response message is shown below
{
"CreateServiceToken_Response_1": {
"Expiration": "2016-09-26T09:42:54.4335265Z",
"Token": "674E12E4EF35F181602672D5529D98379D4B42216057C7FF…"
}
}
IMPLEMENTATION DETAILS
- Firstly, we create a file named “trkd_authen.py” in the working directory. Then we import all required libraries at the top of the source code.
import os
import sys
import requests
import json
import getpass
2. Then we add the code to receive the inputted username, password and application id from the console.
##Get username, password and applicationid
username = input('Please input username: ')
##use getpass.getpass to hide user inputted password
password = getpass.getpass(prompt='Please input password: ')
appid = input('Please input appid: ')
3. Next, we create the request message (authenMsg), the url (authenURL) and headers variables.
##create authentication request URL, message and header
authenMsg = {'CreateServiceToken_Request_1': { 'ApplicationID':appid, 'Username':username,'Password':password }}
authenURL = 'https://api.rkd.refinitiv.com/api/TokenManagement/TokenManagement.svc/REST/Anonymous/TokenManagement_1/CreateServiceToken_1'
headers = {'content-type': 'application/json;charset=utf-8'}
4. Then we create a HTTP Post request message to the RKD Service Token endpoint that specified in the authenURL variable with the authenMsg request message. We use the requests.post function of the requests library to handle this operation.
## send request
result = requests.post(authenURL, data = json.dumps(authenMsg), headers=headers)
5. Finally, we check if the status code is 200 (OK), prints out the Token and Expiration. Alterwise, prints out "request fail message" and raise an exception. If the return status code is 500, prints out the whole return JSON message.
## request success
if result.status_code == 200:
print('Request success')
print('response status %s'%(result.status_code))
## get Token
token = result.json()['CreateServiceToken_Response_1']['Token']
print('Token: %s'%(token))
## get expiraion
expire = result.json()['CreateServiceToken_Response_1']['Expiration']
print('Expire: %s'%(expire))
## handle error
else:
print('Request fail')
print('response status %s'%(result.status_code))
if result.status_code == 500: ## if username or password or appid is wrong
print('Error: %s'%(result.json()))
result.raise_for_status()
Running the application
The application source code is available at GitHub. You can get it via the following git command
$>git clone git@github.com:Refinitiv-API-Samples/Example.TRKD.Python.HTTPJSON.git
$>python trkd_authen.py
Then input your username, password and application id as shown in the Figure-1 below.
Figure-1: The trkd_authen receives username, password and appid via commandline
The result is shown in the Figure-2 below
Figure-2: The Authentication result from RKD Service Token endpoint
Please note that the application must keeps the application id and this service Token for futher operations.
Conclusion
All RKD HTTP JSON applications require authenticaiton to access RKD data. The application needs to request for Service Token from RKD server, then keeps a response Service Token for later use in other request message header.
References
For further details, please check out the following resources:
- Refinitiv Knowledge Direct API page on the Refinitiv Developer Community web site.
- Refinitiv Knowledge Direct API Catalog web site.
For any question related to this article or RKD API, please use the Developer Community Q&A Forum page.